Program that converts a number to a letter of the alphabetProgram that replicates itselfProgram that handles...
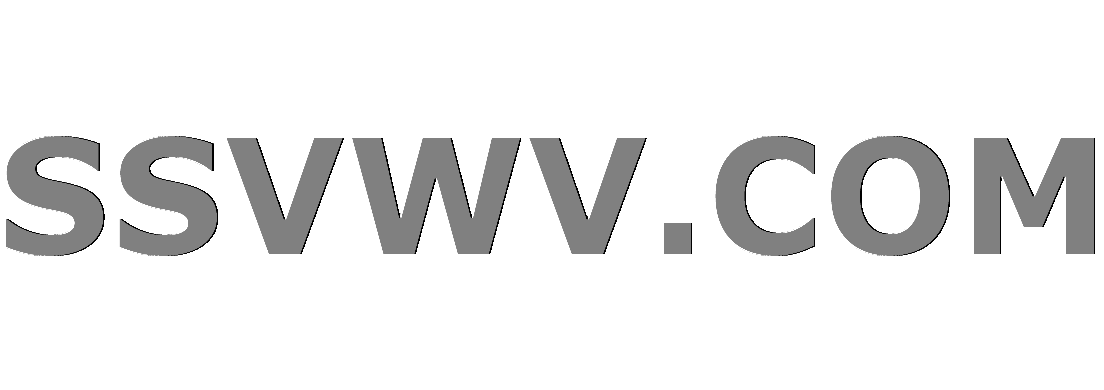
Multi tool use
Am I a Rude Number?
Porting Linux to another platform requirements
Can an insurance company drop you after receiving a bill and refusing to pay?
What is the purpose of easy combat scenarios that don't need resource expenditure?
How to prevent cleaner from hanging my lock screen in Ubuntu 16.04
Roman Numerals equation 1
How long is the D&D Starter Set campaign?
Why is mind meld hard for T'pol in Star Trek: Enterprise?
Why exactly do action photographers need high fps burst cameras?
Is a new Boolean field better than a null reference when a value can be meaningfully absent?
How to limit sight distance to 1 km
Which one of these password policies is more secure?
Can I write a book of my D&D game?
Why publish a research paper when a blog post or a lecture slide can have more citation count than a journal paper?
Why avoid shared user accounts?
My cat mixes up the floors in my building. How can I help him?
Digits in an algebraic irrational number
How would an AI self awareness kill switch work?
Difference between `vector<int> v;` and `vector<int> v = vector<int>();`
Incorporating research and background: How much is too much?
How can animals be objects of ethics without being subjects as well?
Dilemma of explaining to interviewer that he is the reason for declining second interview
Word or phrase for showing great skill at something WITHOUT formal training in it
Can you tell from a blurry photo if focus was too close or too far?
Program that converts a number to a letter of the alphabet
Program that replicates itselfProgram that handles program optionsReverse “Guess the Number” programCustom card gameProgram that guesses your number using bitwise operationsSimple C++ program that calculates the measures of a sphereRandom number distribution programProgram that finds the stretch factor of a parabolaReplace every letter in the string with the letter following it in the alphabet and capitalize vowelsPeter Norvigs' lis.py in c++17
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
#include <iostream>
int main() {
int letter = 0;
std::cout << "Please type in a number: ";
while (1) {
std::cin >> letter;
//less than 1 or greater than 26
if (!(letter < 1 || 26 < letter)) {
std::cout << "The letter that corosponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
std::cout <<"The English Alphabet only has 26 letters. Try againn";
}
}
Gets a integer and prints it as a character. I had thought about using stdio instead of stream but I didn't want it to look scary to a beginner.
c++
c++
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 3 hours ago


Alex AngelAlex Angel
111
111
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Alex Angel is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character’@‘
? Still not obvious what it is doing, or why. How about using the expression ’A’ + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number ”hello”
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and “The English Alphabet ... Try again”
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting “The letter that corosponds [sic] to that value is “
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), ‘n’);
}
std::cout << “Try againn”;
} while (true);
std::cout << “The letter that corresponds ...
$endgroup$
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Alex Angel is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214512%2fprogram-that-converts-a-number-to-a-letter-of-the-alphabet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character’@‘
? Still not obvious what it is doing, or why. How about using the expression ’A’ + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number ”hello”
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and “The English Alphabet ... Try again”
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting “The letter that corosponds [sic] to that value is “
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), ‘n’);
}
std::cout << “Try againn”;
} while (true);
std::cout << “The letter that corresponds ...
$endgroup$
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character’@‘
? Still not obvious what it is doing, or why. How about using the expression ’A’ + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number ”hello”
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and “The English Alphabet ... Try again”
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting “The letter that corosponds [sic] to that value is “
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), ‘n’);
}
std::cout << “Try againn”;
} while (true);
std::cout << “The letter that corresponds ...
$endgroup$
add a comment |
$begingroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character’@‘
? Still not obvious what it is doing, or why. How about using the expression ’A’ + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number ”hello”
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and “The English Alphabet ... Try again”
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting “The letter that corosponds [sic] to that value is “
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), ‘n’);
}
std::cout << “Try againn”;
} while (true);
std::cout << “The letter that corresponds ...
$endgroup$
The declaration int letter = 0;
is perhaps too early in the program. letter
is only used inside the while
loop, so it should be inside of the loop.
Initializing int letter = 0;
is misleading. The initial value of letter
is overwritten by the std::cin >> letter;
statement, so the initial value of 0
is never used, and could be removed.
while (1) {
should be written as while (true) {
. Both amount to an infinite loop, but the latter reads better.
26
is a magic number, so you might want to turn into a named constant. Still, it is a pretty obvious constant, so I’d actually be ok with leaving it as is. However ...
64
is a magic number which absolutely should be turned into a named constant ... or eliminated altogether. What does 64
represent? The ASCII character’@‘
? Still not obvious what it is doing, or why. How about using the expression ’A’ + (letter - 1)
? That is much clearer and better!
Problem: If the user enters the number ”hello”
, the std:cin
stream will go into a fail
state, and executing std::cin >> letter;
subsequent times will continue to fail, and “The English Alphabet ... Try again”
will be repeated forever. You should check for the stream going into the fail
state, clear the error, then discard the invalid input, before returning to reading the next integer.
Outputting “The letter that corosponds [sic] to that value is “
inside the while
loop makes it look like you can enter several values and get the results in the loop, when in reality you can only get one translation before the program exits. You should loop for the user’s input, and break out of the loop once the input is valid, and print the translation outside, after the loop. Something like:
int letter;
do {
if (std::cin >> letter) {
if (letter >= 1 && letter <= 26)
break;
} else {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), ‘n’);
}
std::cout << “Try againn”;
} while (true);
std::cout << “The letter that corresponds ...
answered 1 hour ago
AJNeufeldAJNeufeld
5,4491419
5,4491419
add a comment |
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
add a comment |
$begingroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
$endgroup$
I have five improvements to suggest. The first two are about sanitizing inputs, and are very important. The next two are about user interface design, and are minor. The last is about coding style, and is completely optional.
Fail elegantly on non-integer inputs. This can be done using the
fail()
,clear()
, andignore()
methods ofstd::cin
.Respond to
EOF
appropriately. This can be done usingstd::cin.eof()
.Repeat the prompt after printing an error message.
Check spelling of
"corresponds"
. Do not capitalize"alphabet"
In general, I dislike returning inside of a loop. For a clearer control flow, I would loop until getting a valid input, then, outside the loop convert to a letter.
#include <iostream>
#include <limits>
int main() {
int letter = 0;
while (1) {
std::cout << "Please type in a number: ";
std::cin >> letter;
if (std::cin.eof()) {
std::cout << std::endl;
return 1;
} else if (std::cin.fail()) {
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(),'n');
std::cout <<"Integer input required. Try again.n";
} else if (letter < 1 || letter > 26) {
std::cout <<"The English alphabet only has 26 letters. Try again.n";
} else {
break;
}
}
std::cout << "The letter that corresponds to that value is '"
<< char(64+letter) << "'n";
return 0;
}
answered 1 hour ago
Benjamin KuykendallBenjamin Kuykendall
27014
27014
add a comment |
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
add a comment |
$begingroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
$endgroup$
C++ does not mandate a particular character coding, so while your code is functionally correct on ASCII and ISO-8859 systems, it won't work in EBCDIC environments, for example. Even with the obvious fix to add 'A'-1
in place of the magic constant 64
, the program assumes that English letters have consecutive values, which just isn't the case in BCD encodings.
The portable approach is to index a string literal:
static const std::string_view alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
if (letter > 0 && letter <= alphabet.size()) {
std::cout << "Letter " << letter << " is '"
<< alphabet[letter-1]
<< "'n";
}
Other issues:
- Don't use
letter
before checking whetherstd::cin >> letter
succeeded. - Instead of an infinite loop, prefer a finite loop for reading inputs.
- Consider accepting input as command-line argument(s) as a more convenient alternative to using standard input.
- Spelling: "corresponds".
answered 5 mins ago


Toby SpeightToby Speight
25k741115
25k741115
add a comment |
add a comment |
Alex Angel is a new contributor. Be nice, and check out our Code of Conduct.
Alex Angel is a new contributor. Be nice, and check out our Code of Conduct.
Alex Angel is a new contributor. Be nice, and check out our Code of Conduct.
Alex Angel is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f214512%2fprogram-that-converts-a-number-to-a-letter-of-the-alphabet%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
t3mrJuNJ6 Wpjl9p5s,U418g